Building AI-Powered Applications with Django
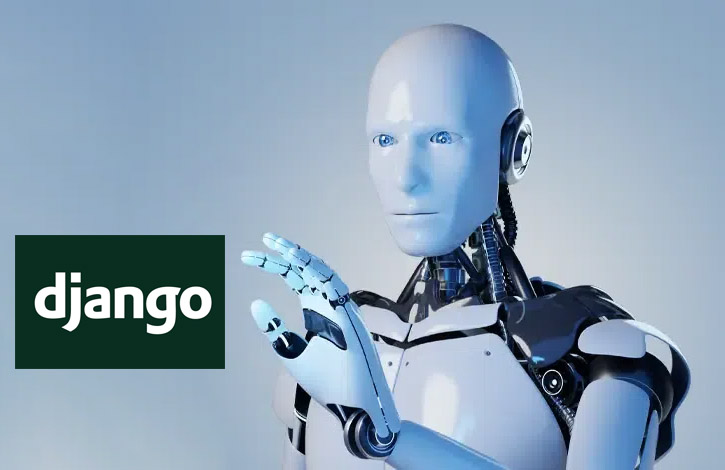
Django has long been a popular choice for web development, thanks to its built-in security, reliability, and extensive community support. Recently, more developers have turned to Django for hosting AI and machine learning (ML) models, capitalizing on its robust architecture to build powerful web applications. In this blog post, we’ll walk through the fundamentals of integrating AI into Django and provide some best practices for deploying advanced analytics and prediction models as part of a modern web ecosystem.
Why Django for AI?
- Scalability: Django's modular architecture allows components such as AI models, databases, and front-end interfaces to scale independently.
- Security: Backed by a large community and fundamental security modules, Django safely handles data from users and ensures a secure environment for sensitive AI pipelines.
- Extensibility: Django integrates smoothly with popular AI frameworks like TensorFlow and PyTorch, making it easier to embed deep learning or traditional ML models into production applications.
- RESTful APIs: With Django REST Framework (DRF), developers can serve AI inferences via REST APIs, enabling cross-platform clients to consume predictions seamlessly.
Setting up the Project
Let’s start by creating a fresh Django project and setting up an environment for AI experimentation. We’ll use a simple example using TensorFlow to load a pre-trained model and serve predictions through Django views.
# 1. Create and activate a virtual environment python -m venv myenv source myenv/bin/activate # or myenv\Scripts\activate on Windows # 2. Install Django, TensorFlow, and Django REST Framework (optional, for APIs) pip install django tensorflow djangorestframework # 3. Initialize a new Django project django-admin startproject ai_project cd ai_project # 4. Create a new Django app python manage.py startapp ai_app
Loading and Integrating the Model
Suppose we have a pre-trained TensorFlow model saved in the .h5 format. We’ll load that model in our ai_app and set up a view to handle user input for prediction.
# ai_app/models.py # Not to be confused with Django's built-in "models" for databases; # this file can hold your AI model code. import tensorflow as tf model = tf.keras.models.load_model('path/to/your_model.h5') def predict(input_data): # input_data should be preprocessed to match the model's input shape prediction = model.predict(input_data) return prediction
Next, we'll create a view that utilizes this TensorFlow model to produce predictions. If you're using Django REST Framework, you can create an API endpoint that accepts user data and returns the predicted results.
# ai_app/views.py from django.http import JsonResponse, HttpResponseBadRequest from .models import predict import numpy as np def infer_view(request): if request.method == 'POST': user_input = request.POST.get('user_input') if not user_input: return HttpResponseBadRequest('No input data provided.') # Example: converting user_input into a 2D numpy array # In a real scenario, handle input parsing and preprocessing carefully input_array = np.array([[float(x) for x in user_input.split(",")]]) prediction_result = predict(input_array) # Convert numpy array to list for JSON serialization prediction_list = prediction_result.tolist() return JsonResponse({'prediction': prediction_list}) else: return HttpResponseBadRequest('Invalid request method.')
URL Configuration
To map the infer_view to a URL, add a route in ai_app/urls.py (remember to include these urls in your ai_project/urls.py).
# ai_app/urls.py from django.urls import path from .views import infer_view urlpatterns = [ path('infer/', infer_view, name='infer_view'), ]
Running Your AI-powered Django App
With everything in place, you can now run the server and test out your AI endpoint locally:
python manage.py makemigrations python manage.py migrate python manage.py runserver
Once the server is running, you can make a POST request to /infer/ with data to receive predictions. If you’re using a REST client (like Postman or cURL), send a form data field named user_input containing comma-separated values. The server will respond with a JSON object containing the prediction.
Best Practices for AI in Django
- Model Caching: If your model is large or if you are repeatedly loading the same model, consider loading it once at server startup. This approach cuts down on model loading overhead for each request.
- Batch Processing: For high-traffic scenarios, consider grouping requests into batches, feeding them into ML models in bulk to leverage parallel processing capabilities of frameworks like TensorFlow.
- Model Updates: If your AI system needs to be retrained or updated, incorporate a reliable pipeline or continuous integration mechanism to rebuild and reload models with zero downtime or minimal interruption.
- Monitoring and Logging: AI-based applications are data hungry. Track incoming requests, predictions, performance metrics (time taken, resource usage), and error rates to ensure your model continues to behave as expected.
Conclusion
Adding AI capabilities to your Django application can open doors to innovative features and interactive user experiences. Thanks to the framework’s flexibility and rich ecosystem, you can integrate machine learning models, preprocess incoming data, and deliver results to users swiftly. Whether you’re creating a data-driven startup or enhancing an existing product, Django provides a solid foundation to build and scale AI-powered web applications.
We hope this blog post helps you get started with integrating AI in Django. Feel free to explore more sophisticated approaches like streaming, containerization, or advanced GPU-assisted backends to take your application to the next level!
Django used for AI.
Repost Disclaimer: This content is copyrighted and all rights are reserved by the author. You are welcome to repost or share this page, but please ensure you provide a clear credit to the original source with a hyperlink back to this page. Thank you for respecting our content!
Was this article helpful?
5526 out of 5531 found this helpful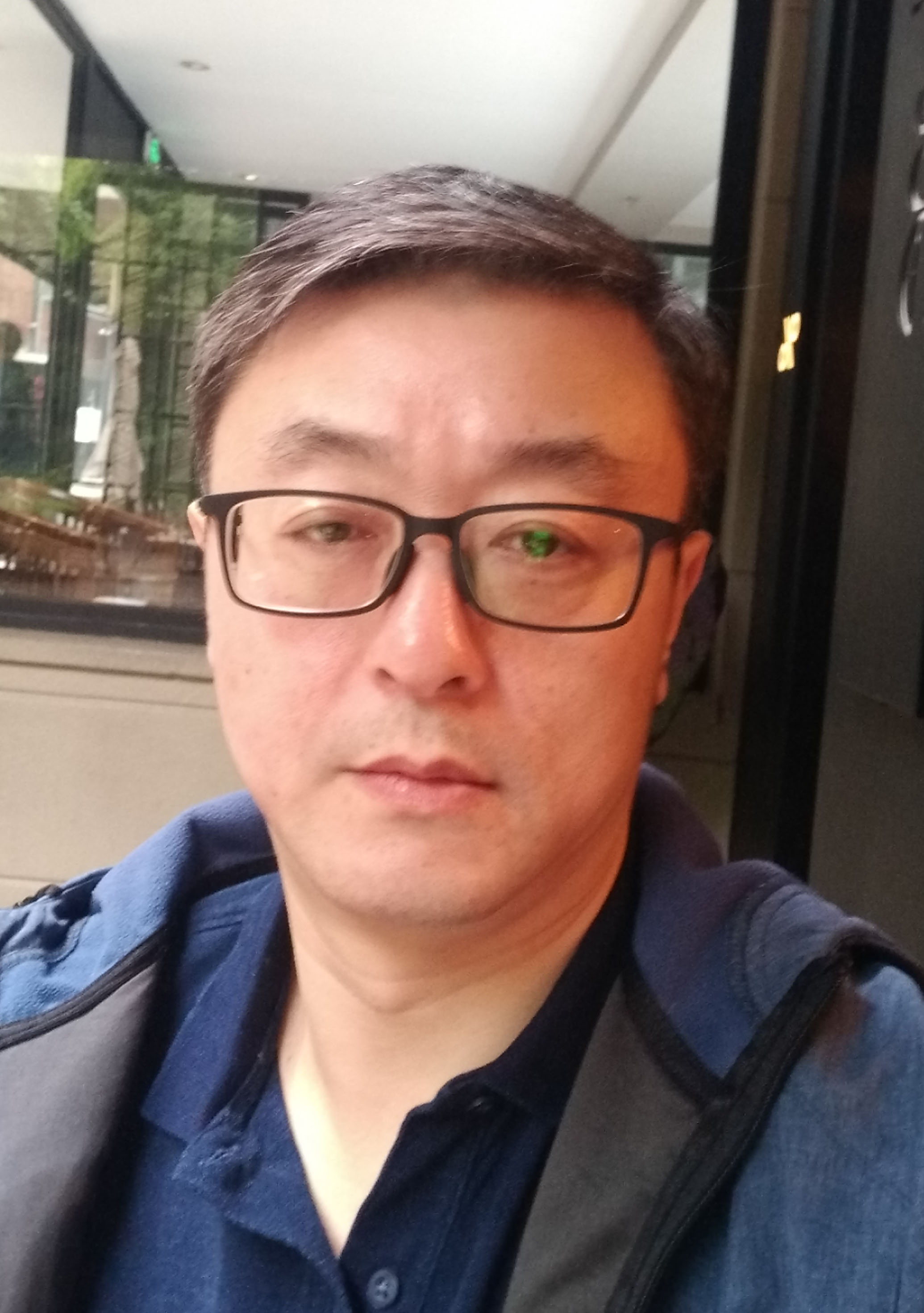
John Tanner
FounderI am a highly skilled software developer with over 20 years of experience in cross-platform full-stack development. I specialize in designing and managing large-scale project architectures and simplifying complex systems. My expertise extends to Python, Rust, and Django development. I have a deep proficiency in blockchain technologies, artificial intelligence, high concurrency systems, app and web data scraping, API development, database optimization, containerizing projects, and deploying in production environments.
Contact John TannerRelated post:
- Scheduling Asynchronous Multithreaded Tasks in Django with Celery
- How ChatGPT Can Revolutionize Multilingual Customer Service: A Comprehensive Guide
- How to Build a Successful Overseas Independent Website for Foreign Trade: A Comprehensive Guide from Market Research to SEO Optimization
- Building a Multilingual Website with Django: Best Practices and SEO Strategies
- Unveiling the Power of Django: The Ultimate Backend Framework