Scheduling Asynchronous Multithreaded Tasks in Django with Celery
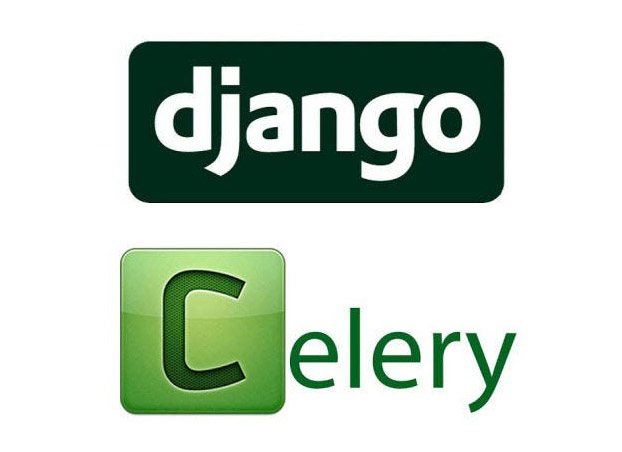
Managing tasks in a web application can become complex, especially when you need to perform time-consuming operations asynchronously. Django, a popular web framework, can be extended with Celery to handle asynchronous tasks efficiently. In this blog post, we're going to explore how to set up periodic task scheduling with Celery in a Django application. This allows your application to run background tasks at specified intervals and offload task processing from the main application threads.
1. Install Celery and Django Celery Beat
The journey begins with installing Celery and django-celery-beat. These are essential components for task scheduling and execution in Django. You can install them using pip:
pip install celery django-celery-beat
2. Configure Celery
After installing Celery, you need to configure it to work with your Django application. Start by creating a file named celery.py in your project's main directory. This file will initialize Celery and link it to Django's settings.
from celery import Celery import os # Set the default Django settings module for the 'celery' program. os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'your_project_name.settings') app = Celery('your_project_name') # Using a string here means the worker will not have to serialize # the configuration object to child processes. app.config_from_object('django.conf:settings', namespace='CELERY') # Load task modules from all registered Django apps. app.autodiscover_tasks()
To ensure this configuration is loaded when Django starts, import your Celery app in the your_project_name/__init__.py file:
from .celery import app as celery_app __all__ = ('celery_app',)
3. Update Django Settings
Next, integrate Celery with your Django settings by updating the settings.py file. This includes specifying your broker and result backend, which could be RabbitMQ or Redis.
CELERY_BROKER_URL = 'redis://localhost:6379/0' # or 'amqp://localhost//' for RabbitMQ CELERY_RESULT_BACKEND = 'redis://localhost:6379/0' CELERY_TIMEZONE = 'UTC' from celery.schedules import crontab CELERY_BEAT_SCHEDULE = { 'task-name': { 'task': 'myapp.tasks.task_function', 'schedule': crontab(minute=0, hour=0), # Executes daily at midnight }, }
4. Define Your Task
Now, it's time to define what your tasks should be. Create a tasks.py file in your Django app (e.g., myapp ) and add your task function.
from celery import shared_task @shared_task def task_function(): # Logic for your asynchronous, multithreaded task print("Task is executing")
Here, @shared_task is a decorator provided by Celery that marks the function to be a task callable.
5. Run Celery Worker and Beat
Finally, you need to run the Celery worker and beat to start processing the tasks and scheduling them according to your configuration.
# Start Celery Worker celery -A your_project_name worker -l info # Start Celery Beat celery -A your_project_name beat -l info
These commands launch the worker to process asynchronous tasks and the beat process to manage periodic tasks. Ensure your message broker like Redis or RabbitMQ is running before executing these commands.
By following these steps, you've equipped your Django application to efficiently handle periodic asynchronous tasks, enhancing the performance and responsiveness of your application. Celery is a powerful tool, and with this setup, you're ready to explore more complex task processing ideas.
Repost Disclaimer: This content is copyrighted and all rights are reserved by the author. You are welcome to repost or share this page, but please ensure you provide a clear credit to the original source with a hyperlink back to this page. Thank you for respecting our content!
Was this article helpful?
196 out of 196 found this helpful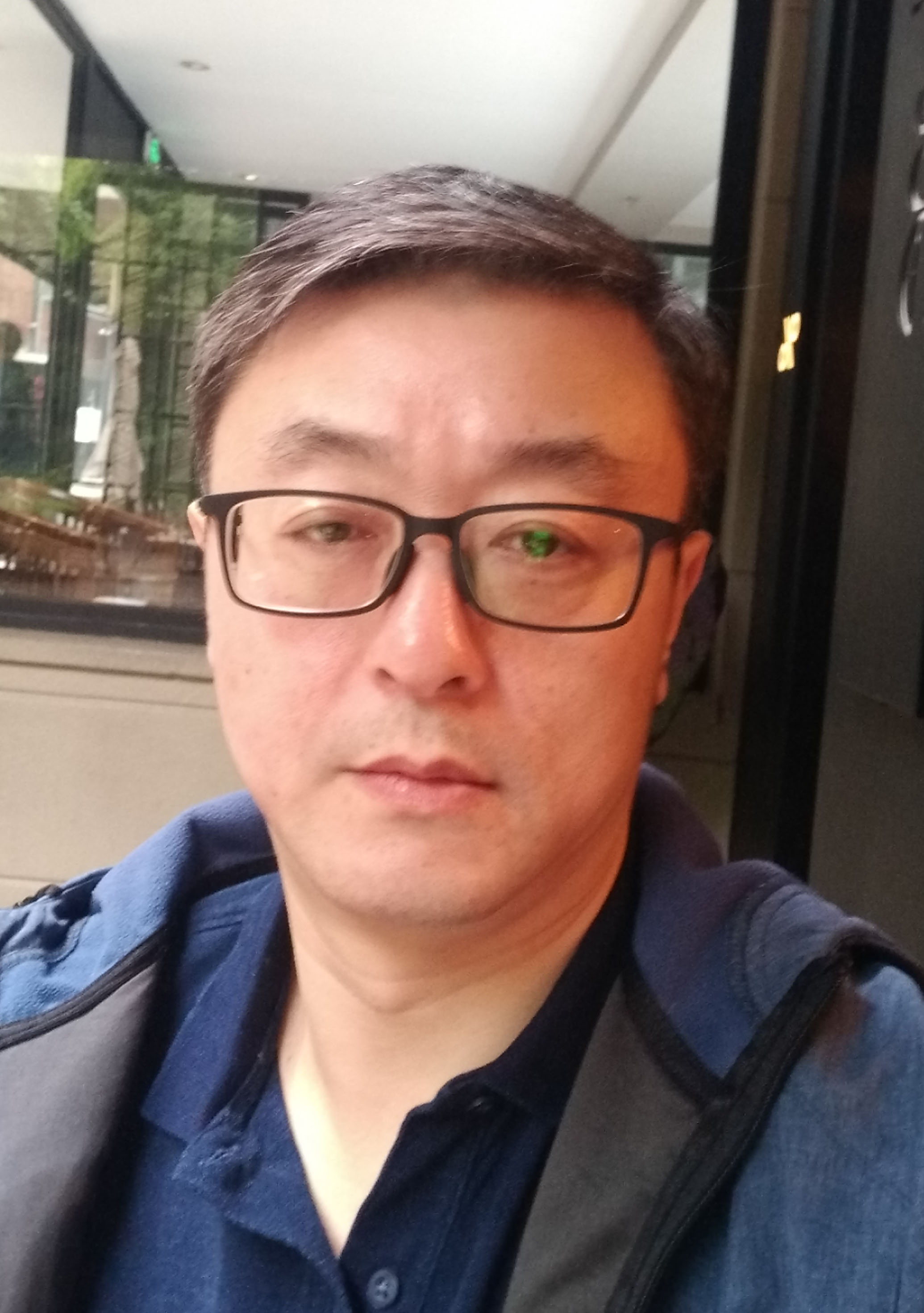
John Tanner
FounderI am a highly skilled software developer with over 20 years of experience in cross-platform full-stack development. I specialize in designing and managing large-scale project architectures and simplifying complex systems. My expertise extends to Python, Rust, and Django development. I have a deep proficiency in blockchain technologies, artificial intelligence, high concurrency systems, app and web data scraping, API development, database optimization, containerizing projects, and deploying in production environments.
Contact John TannerRelated post:
- Building a Multilingual Website with Django: Best Practices and SEO Strategies
- How to Build a Successful Overseas Independent Website for Foreign Trade: A Comprehensive Guide from Market Research to SEO Optimization
- Django Development Services
- Building AI-Powered Applications with Django
- Unveiling the Power of Django: The Ultimate Backend Framework